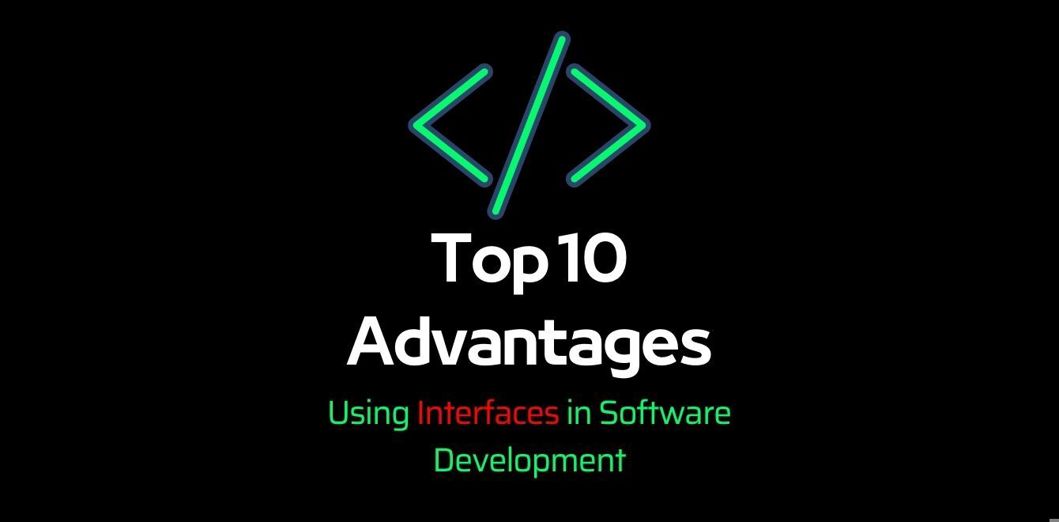
There are many reasons why interfaces are widely used in software development. Here are the top 10 reasons, along with examples to illustrate each use case.
1. Abstraction:
Interfaces allow you to define a contract that classes must adhere to, promoting abstraction and hiding implementation details. For example, an IVehicle interface can define methods like Start(), Stop(), and Drive() that different vehicle classes can implement.
2. Code Reusability:
Interfaces enable code reuse by providing a common set of methods that multiple classes can implement. For instance, an IList interface can define methods like Add(), Remove(), and Count(), which can be implemented by various list classes such as ArrayList, LinkedList, and SortedSet.
3. Polymorphism:
Interfaces facilitate polymorphism, allowing objects of different classes that implement the same interface to be treated uniformly. This enables flexible and extensible code. For example, you can have a Print() method that accepts any object implementing the IPrintable interface and can print different types of printable objects.
4. Dependency Injection:
Interfaces are commonly used in dependency injection, where objects depend on abstractions rather than concrete implementations. By injecting dependencies through interfaces, you can easily swap implementations at runtime. For instance, you can inject different ILogger implementations into a LogManager class without changing its code.
5. Testing and Mocking:
Interfaces facilitate unit testing by allowing you to create mock objects that simulate the behavior of dependencies. With interfaces, you can easily provide mock implementations for testing purposes, ensuring isolated and controlled testing scenarios.
6. Multiple Inheritance-like Behavior:
C# doesn't support multiple inheritance, but interfaces can emulate similar behavior. By implementing multiple interfaces, a class can inherit and provide different sets of behaviors. For example, a class can implement both IFlyable and ISwimmable interfaces to exhibit both flying and swimming behavior.
7. Separation of Concerns:
Interfaces help in separating concerns by defining specific contracts for different aspects of a system. For example, an IPersistenceService interface can define methods for data persistence, allowing the actual persistence logic to be implemented separately by different classes.
8. Plugin Architecture:
Interfaces are useful for creating plugin architectures, where external modules can be dynamically loaded and integrated into an application. The interfaces define the contract that plugins must adhere to, allowing for extensible and customizable applications.
9. API Design:
Interfaces play a crucial role in designing APIs by defining the contracts and expected behaviors for clients. For example, a payment gateway API might define an IPaymentGateway interface that specifies methods like ProcessPayment() and RefundPayment().
10. Loose Coupling:
Interfaces promote loose coupling between components, reducing dependencies on concrete implementations. This improves maintainability and allows for easier future changes. For instance, you can have a IDatabaseConnector interface that decouples your code from specific database providers, enabling you to switch between databases without major code modifications.
Interface provides flexibility, modularity, code reusability, and facilitate better design and problem-solving practices.